Can we inherit a final method in Java?
I'll answer
Earn 20 gold coins for an accepted answer.20
Earn 20 gold coins for an accepted answer.
40more
40more
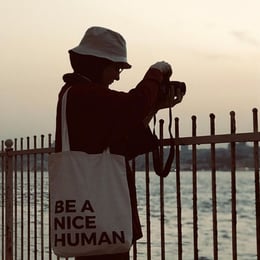
Scarlett Gonzales
Studied at the University of British Columbia, Lives in Vancouver, Canada.
As an expert in the field of computer science with a focus on Java, I'd like to clarify some common misconceptions regarding the use of the `final` keyword in the context of inheritance. The `final` keyword in Java serves multiple purposes and its use can be nuanced depending on the context. Let's delve into the details of how `final` can be applied to methods and classes, and what it means for inheritance.
Firstly, it's important to understand what the `final` keyword signifies when applied to different elements of a Java program:
1. Final Variables: When a variable is declared final, it means that once a value is assigned to it, that value cannot be changed. This is often used for constants.
2. Final Methods: A final method is one that cannot be overridden by subclasses. This means that the implementation of the method is fixed and must be used as is by any subclass.
3. Final Classes: A final class cannot be subclassed. No other class can inherit from a final class.
Now, let's focus on the use of the `final` keyword with inheritance:
- When you declare a method as final in a superclass, you are indicating that any subclass must use the exact implementation of that method and cannot provide its own. This is useful when you want to ensure that a particular behavior is consistent across all subclasses and cannot be altered.
- It's a common mistake to think that you must declare methods as final in order to prevent them from being overridden. However, in Java, if a method is not explicitly declared as final, it is by default not final, and thus can be overridden by subclasses, unless it is a private method, which cannot be overridden due to accessibility constraints rather than the `final` keyword.
- Declaring a class as final prevents any other class from inheriting from it. This is often done when the class is complete and the designer does not want it to be extended.
- It's also worth noting that Java allows you to use the `final` keyword with constructors. A final constructor prevents subclassing because there would be no way to call a superclass's constructor if it is final.
Here's an example to illustrate the use of the `final` keyword with inheritance:
```java
public class BaseClass {
public final void show() {
System.out.println("This method cannot be overridden");
}
public void changeableMethod() {
System.out.println("This method can be overridden");
}
}
public class SubClass extends BaseClass {
@Override
public void changeableMethod() {
System.out.println("Overridden method in subclass");
}
// The following line would cause a compile error because 'show' is final in BaseClass
// @Override
// public void show() {
// System.out.println("Cannot override final method");
// }
}
```
In the example above, the `show` method in `BaseClass` is final and cannot be overridden in `SubClass`, while the `changeableMethod` is not final and can be overridden.
To summarize, the `final` keyword is a powerful tool in Java that can be used to control the behavior of variables, methods, and classes with respect to inheritance. It is not mandatory to declare methods as final to prevent them from being overridden unless you want to ensure that the method's implementation remains unchanged in all subclasses. Understanding when and why to use `final` is crucial for writing robust and maintainable Java code.
Firstly, it's important to understand what the `final` keyword signifies when applied to different elements of a Java program:
1. Final Variables: When a variable is declared final, it means that once a value is assigned to it, that value cannot be changed. This is often used for constants.
2. Final Methods: A final method is one that cannot be overridden by subclasses. This means that the implementation of the method is fixed and must be used as is by any subclass.
3. Final Classes: A final class cannot be subclassed. No other class can inherit from a final class.
Now, let's focus on the use of the `final` keyword with inheritance:
- When you declare a method as final in a superclass, you are indicating that any subclass must use the exact implementation of that method and cannot provide its own. This is useful when you want to ensure that a particular behavior is consistent across all subclasses and cannot be altered.
- It's a common mistake to think that you must declare methods as final in order to prevent them from being overridden. However, in Java, if a method is not explicitly declared as final, it is by default not final, and thus can be overridden by subclasses, unless it is a private method, which cannot be overridden due to accessibility constraints rather than the `final` keyword.
- Declaring a class as final prevents any other class from inheriting from it. This is often done when the class is complete and the designer does not want it to be extended.
- It's also worth noting that Java allows you to use the `final` keyword with constructors. A final constructor prevents subclassing because there would be no way to call a superclass's constructor if it is final.
Here's an example to illustrate the use of the `final` keyword with inheritance:
```java
public class BaseClass {
public final void show() {
System.out.println("This method cannot be overridden");
}
public void changeableMethod() {
System.out.println("This method can be overridden");
}
}
public class SubClass extends BaseClass {
@Override
public void changeableMethod() {
System.out.println("Overridden method in subclass");
}
// The following line would cause a compile error because 'show' is final in BaseClass
// @Override
// public void show() {
// System.out.println("Cannot override final method");
// }
}
```
In the example above, the `show` method in `BaseClass` is final and cannot be overridden in `SubClass`, while the `changeableMethod` is not final and can be overridden.
To summarize, the `final` keyword is a powerful tool in Java that can be used to control the behavior of variables, methods, and classes with respect to inheritance. It is not mandatory to declare methods as final to prevent them from being overridden unless you want to ensure that the method's implementation remains unchanged in all subclasses. Understanding when and why to use `final` is crucial for writing robust and maintainable Java code.
2024-05-12 12:10:13
reply(1)
Helpful(1122)
Helpful
Helpful(2)
Studied at Harvard University, Lives in Boston. Passionate about environmental conservation and currently working for a non-profit organization.
Using final with Inheritance in Java. ... We can declare variables, methods and classes with final keyword. Using final with inheritance. During inheritance, we must declare methods with final keyword for which we required to follow the same implementation throughout all the derived classes.
2023-06-10 06:42:40

Lily Patel
QuesHub.com delivers expert answers and knowledge to you.
Using final with Inheritance in Java. ... We can declare variables, methods and classes with final keyword. Using final with inheritance. During inheritance, we must declare methods with final keyword for which we required to follow the same implementation throughout all the derived classes.