Can we have more than one main method in Java?
I'll answer
Earn 20 gold coins for an accepted answer.20
Earn 20 gold coins for an accepted answer.
40more
40more

Olivia Garcia
Studied at Harvard University, Lives in Cambridge, MA
Hello! As an expert in the field of software development, I'm here to provide you with a detailed explanation regarding your question about Java's main method.
In Java, every application must contain a main method. This method serves as the entry point for the program, and it is the first method that is executed when the program starts. However, there's a common misconception that there can only be one main method in a Java application. Let's clarify this.
### The Main Method in Java
The main method is a special method in Java that has the following signature:
```java
public static void main(String[] args)
```
Here's what each part of the signature means:
- public: This means that the method is accessible from any other class.
- static: This means that the method can be called without creating an instance of the class it is defined in.
- void: This means that the method does not return any value.
- main: This is the name of the method.
- String[] args: This is the parameter that the method takes, an array of strings that can be used to pass command-line arguments to the program.
### Multiple Classes with Main Methods
It is true that in Java, you can have just one public static void main(String[] args) per class. However, this does not mean that you can only have one main method in your entire program. If your program has multiple classes, each class can define its own main method. This is useful in several scenarios:
1. Separation of Concerns: You can separate different functionalities into different classes and have a main method in each class to test or run those functionalities independently.
2. Modularity: It allows for better modularity, as each class can be developed and tested independently.
3. Reusability: Classes can be reused in different contexts without the need to modify the main method.
### Defining Multiple Main Methods within a Class
While it's true that a class can only have one public static void main(String[] args) method, you can define multiple methods with the name "main" as long as they have different parameter lists (overloading). For example:
```java
public class MyClass {
public static void main(String[] args) {
// First main method
}
public static void main(String[] arg) { // Note the different parameter name
// Second main method with a different parameter list
}
}
```
However, only one of these methods can be the entry point for the program. The one that matches the required signature for the main method (`public static void main(String[] args)`) will be used by the Java Virtual Machine (JVM) as the program's entry point.
### Conclusion
To sum up, while each class in Java can only have one public static void main(String[] args) method, you can have multiple classes, each with its own main method. This allows for a flexible and modular approach to program design. It's important to note that only one of these main methods will serve as the entry point for the entire application when it is run.
Now, let's move on to the translation of the explanation into Chinese.
In Java, every application must contain a main method. This method serves as the entry point for the program, and it is the first method that is executed when the program starts. However, there's a common misconception that there can only be one main method in a Java application. Let's clarify this.
### The Main Method in Java
The main method is a special method in Java that has the following signature:
```java
public static void main(String[] args)
```
Here's what each part of the signature means:
- public: This means that the method is accessible from any other class.
- static: This means that the method can be called without creating an instance of the class it is defined in.
- void: This means that the method does not return any value.
- main: This is the name of the method.
- String[] args: This is the parameter that the method takes, an array of strings that can be used to pass command-line arguments to the program.
### Multiple Classes with Main Methods
It is true that in Java, you can have just one public static void main(String[] args) per class. However, this does not mean that you can only have one main method in your entire program. If your program has multiple classes, each class can define its own main method. This is useful in several scenarios:
1. Separation of Concerns: You can separate different functionalities into different classes and have a main method in each class to test or run those functionalities independently.
2. Modularity: It allows for better modularity, as each class can be developed and tested independently.
3. Reusability: Classes can be reused in different contexts without the need to modify the main method.
### Defining Multiple Main Methods within a Class
While it's true that a class can only have one public static void main(String[] args) method, you can define multiple methods with the name "main" as long as they have different parameter lists (overloading). For example:
```java
public class MyClass {
public static void main(String[] args) {
// First main method
}
public static void main(String[] arg) { // Note the different parameter name
// Second main method with a different parameter list
}
}
```
However, only one of these methods can be the entry point for the program. The one that matches the required signature for the main method (`public static void main(String[] args)`) will be used by the Java Virtual Machine (JVM) as the program's entry point.
### Conclusion
To sum up, while each class in Java can only have one public static void main(String[] args) method, you can have multiple classes, each with its own main method. This allows for a flexible and modular approach to program design. It's important to note that only one of these main methods will serve as the entry point for the entire application when it is run.
Now, let's move on to the translation of the explanation into Chinese.
2024-05-12 12:10:18
reply(1)
Helpful(1122)
Helpful
Helpful(2)
Studied at the University of Ottawa, Lives in Ottawa, Canada.
In Java, you can have just one public static void main(String[] args) per class. Which mean, if your program has multiple classes, each class can have public static void main(String[] args) . See JLS for details. A class can define multiple methods with the name main.Nov 15, 2012
2023-06-10 06:42:39
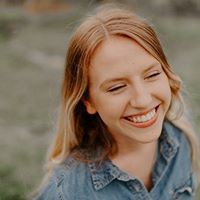
Charlotte Cooper
QuesHub.com delivers expert answers and knowledge to you.
In Java, you can have just one public static void main(String[] args) per class. Which mean, if your program has multiple classes, each class can have public static void main(String[] args) . See JLS for details. A class can define multiple methods with the name main.Nov 15, 2012