Can a static method be inherited?
I'll answer
Earn 20 gold coins for an accepted answer.20
Earn 20 gold coins for an accepted answer.
40more
40more
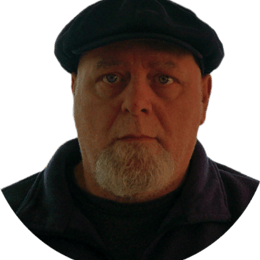
Benjamin Adams
Works at Amazon, Lives in Seattle. Graduated from University of Washington with a degree in Business Administration.
Hello, I'm a seasoned expert in the field of computer science with a focus on programming languages and object-oriented design. I'm here to help you understand the intricacies of static methods and their behavior in inheritance.
When we discuss inheritance in object-oriented programming (OOP), we're referring to the process by which one class can inherit properties and behaviors (methods) from another class. This is a fundamental concept that allows for code reuse and the creation of a hierarchy of classes. However, not all elements of a class are subject to the same inheritance rules.
In Java, static methods are indeed inherited by subclasses. This means that if a class defines a static method, any subclass that is derived from that class will have access to that static method. However, the inheritance of static methods is different from the inheritance of instance methods in several key ways:
1. Accessibility: A static method in a superclass can be accessed by a subclass regardless of the access modifier (public, protected, or private) of the static method in the superclass. This is because static methods are associated with the class itself, not instances of the class.
2. Polymorphism: Static methods do not participate in polymorphism. Polymorphism allows for methods to be overridden in subclasses to provide specific behavior. When you override an instance method, a call to that method on an object of the subclass will invoke the subclass's version of the method, not the superclass's. This is not the case with static methods. If you attempt to override a static method in a subclass, you are not actually overriding it; instead, you are hiding the method from the superclass. This means that a call to the static method will invoke the version that is determined by the static type of the reference used, not the dynamic type of the object.
3. Method Hiding: In the case of static methods, what happens is known as method hiding, not overriding. If a subclass has a static method with the same signature as a static method in its superclass, the subclass's method will be used when it is accessed through a reference to the subclass. However, this is not considered polymorphism because the decision of which method to invoke is made at compile-time, not runtime.
4. Invocation: Static methods are invoked using the class name, not an instance of the class. This is a clear indication that they are not tied to any particular instance and thus do not have the same dynamic behavior as instance methods.
Here's an example to illustrate the concept:
```java
public class Superclass {
public static void staticMethod() {
System.out.println("Superclass static method");
}
}
public class Subclass extends Superclass {
public static void staticMethod() {
System.out.println("Subclass static method");
}
}
public class Test {
public static void main(String[] args) {
Superclass.staticMethod(); // Outputs: Superclass static method
Subclass.staticMethod(); // Outputs: Subclass static method
// The following line will not compile because the method is static and
// there's no object to invoke it on.
// Superclass obj = new Superclass();
// obj.staticMethod();
}
}
```
In the example above, the `staticMethod` in `Subclass` hides the `staticMethod` in `Superclass`. If you call `Subclass.staticMethod()`, it will execute the version in `Subclass`, but this is not an example of polymorphism.
To summarize, while static methods are inherited in the sense that they are available to subclasses, they do not exhibit polymorphic behavior. When you have a static method in a subclass with the same signature as one in the superclass, you are not overriding the method; you are hiding it. This is an important distinction to understand when working with static methods in Java.
When we discuss inheritance in object-oriented programming (OOP), we're referring to the process by which one class can inherit properties and behaviors (methods) from another class. This is a fundamental concept that allows for code reuse and the creation of a hierarchy of classes. However, not all elements of a class are subject to the same inheritance rules.
In Java, static methods are indeed inherited by subclasses. This means that if a class defines a static method, any subclass that is derived from that class will have access to that static method. However, the inheritance of static methods is different from the inheritance of instance methods in several key ways:
1. Accessibility: A static method in a superclass can be accessed by a subclass regardless of the access modifier (public, protected, or private) of the static method in the superclass. This is because static methods are associated with the class itself, not instances of the class.
2. Polymorphism: Static methods do not participate in polymorphism. Polymorphism allows for methods to be overridden in subclasses to provide specific behavior. When you override an instance method, a call to that method on an object of the subclass will invoke the subclass's version of the method, not the superclass's. This is not the case with static methods. If you attempt to override a static method in a subclass, you are not actually overriding it; instead, you are hiding the method from the superclass. This means that a call to the static method will invoke the version that is determined by the static type of the reference used, not the dynamic type of the object.
3. Method Hiding: In the case of static methods, what happens is known as method hiding, not overriding. If a subclass has a static method with the same signature as a static method in its superclass, the subclass's method will be used when it is accessed through a reference to the subclass. However, this is not considered polymorphism because the decision of which method to invoke is made at compile-time, not runtime.
4. Invocation: Static methods are invoked using the class name, not an instance of the class. This is a clear indication that they are not tied to any particular instance and thus do not have the same dynamic behavior as instance methods.
Here's an example to illustrate the concept:
```java
public class Superclass {
public static void staticMethod() {
System.out.println("Superclass static method");
}
}
public class Subclass extends Superclass {
public static void staticMethod() {
System.out.println("Subclass static method");
}
}
public class Test {
public static void main(String[] args) {
Superclass.staticMethod(); // Outputs: Superclass static method
Subclass.staticMethod(); // Outputs: Subclass static method
// The following line will not compile because the method is static and
// there's no object to invoke it on.
// Superclass obj = new Superclass();
// obj.staticMethod();
}
}
```
In the example above, the `staticMethod` in `Subclass` hides the `staticMethod` in `Superclass`. If you call `Subclass.staticMethod()`, it will execute the version in `Subclass`, but this is not an example of polymorphism.
To summarize, while static methods are inherited in the sense that they are available to subclasses, they do not exhibit polymorphic behavior. When you have a static method in a subclass with the same signature as one in the superclass, you are not overriding the method; you are hiding it. This is an important distinction to understand when working with static methods in Java.
2024-05-12 21:18:11
reply(1)
Helpful(1122)
Helpful
Helpful(2)
Studied at the University of Buenos Aires, Lives in Buenos Aires, Argentina.
Static methods are inherited in Java but they don't take part in polymorphism. If we attempt to override the static methods they will just hide the superclass static methods instead of overriding them. Static method is inherited in subclass but it is not polymorphism.Apr 24, 2012
2023-06-14 06:42:28

Owen Turner
QuesHub.com delivers expert answers and knowledge to you.
Static methods are inherited in Java but they don't take part in polymorphism. If we attempt to override the static methods they will just hide the superclass static methods instead of overriding them. Static method is inherited in subclass but it is not polymorphism.Apr 24, 2012