Can we extend an interface in class?
I'll answer
Earn 20 gold coins for an accepted answer.20
Earn 20 gold coins for an accepted answer.
40more
40more

Lucas Jackson
Works at the International Development Association, Lives in Washington, D.C., USA.
As an expert in the field of software development, particularly in object-oriented programming (OOP), I often encounter questions regarding the use and extension of interfaces and classes. Let's delve into the concept of extending an interface within a class in the context of Java, which is a common language where such a question might arise.
In object-oriented programming, an interface is a collection of abstract methods that define a set of functionality. It is a contract that specifies what methods a class must implement, but not how those methods are implemented. On the other hand, an abstract class is a class that cannot be instantiated on its own and is meant to be subclassed. It can contain both abstract methods (without an implementation) and concrete methods (with an implementation).
Now, let's address the question of extending an interface within a class. In Java, an interface can indeed be "extended" by another interface, but this is done through the keyword `extends`, similar to how a class extends another class. However, when we talk about implementing an interface in a class, we use the keyword `implements`. Here's the distinction:
1. Extending an Interface: When one interface extends another, it inherits the abstract methods of the parent interface and can also define additional abstract methods. This is useful for creating a hierarchy of interfaces.
2. Implementing an Interface in a Class: A class implements an interface by providing concrete implementations for all the abstract methods defined in the interface. A class can implement multiple interfaces, but it can extend only one class (due to Java's single inheritance limitation).
Regarding the statement "An abstract class is still a class, just like any other. Only interfaces can extend other interfaces.", this is partially correct. While it is true that only interfaces can extend other interfaces, an abstract class can implement one or more interfaces. This allows the abstract class to inherit the contract of the interface(s) and provide some default behavior if desired.
Here's an example to illustrate this:
```java
interface FeatureA {
void doSomething();
}
interface FeatureB extends FeatureA {
void doSomethingElse();
}
abstract class MyClass implements FeatureB {
public void doSomething() {
// Default implementation
}
public void doSomethingElse() {
// Another default implementation
}
// Additional methods and fields can be added here
}
class SubClass extends MyClass {
// SubClass inherits all methods from MyClass and must provide its own implementation if needed
}
```
In this example, `FeatureB` extends `FeatureA`, creating an interface hierarchy. `MyClass` is an abstract class that implements `FeatureB`, providing default behavior for the methods defined in the interfaces.
To summarize, while you cannot extend an interface with a class directly, you can have a class implement an interface, and an abstract class can also implement one or more interfaces. This provides a way to achieve polymorphism and code reusability in Java.
In object-oriented programming, an interface is a collection of abstract methods that define a set of functionality. It is a contract that specifies what methods a class must implement, but not how those methods are implemented. On the other hand, an abstract class is a class that cannot be instantiated on its own and is meant to be subclassed. It can contain both abstract methods (without an implementation) and concrete methods (with an implementation).
Now, let's address the question of extending an interface within a class. In Java, an interface can indeed be "extended" by another interface, but this is done through the keyword `extends`, similar to how a class extends another class. However, when we talk about implementing an interface in a class, we use the keyword `implements`. Here's the distinction:
1. Extending an Interface: When one interface extends another, it inherits the abstract methods of the parent interface and can also define additional abstract methods. This is useful for creating a hierarchy of interfaces.
2. Implementing an Interface in a Class: A class implements an interface by providing concrete implementations for all the abstract methods defined in the interface. A class can implement multiple interfaces, but it can extend only one class (due to Java's single inheritance limitation).
Regarding the statement "An abstract class is still a class, just like any other. Only interfaces can extend other interfaces.", this is partially correct. While it is true that only interfaces can extend other interfaces, an abstract class can implement one or more interfaces. This allows the abstract class to inherit the contract of the interface(s) and provide some default behavior if desired.
Here's an example to illustrate this:
```java
interface FeatureA {
void doSomething();
}
interface FeatureB extends FeatureA {
void doSomethingElse();
}
abstract class MyClass implements FeatureB {
public void doSomething() {
// Default implementation
}
public void doSomethingElse() {
// Another default implementation
}
// Additional methods and fields can be added here
}
class SubClass extends MyClass {
// SubClass inherits all methods from MyClass and must provide its own implementation if needed
}
```
In this example, `FeatureB` extends `FeatureA`, creating an interface hierarchy. `MyClass` is an abstract class that implements `FeatureB`, providing default behavior for the methods defined in the interfaces.
To summarize, while you cannot extend an interface with a class directly, you can have a class implement an interface, and an abstract class can also implement one or more interfaces. This provides a way to achieve polymorphism and code reusability in Java.
2024-05-12 12:11:05
reply(1)
Helpful(1122)
Helpful
Helpful(2)
Studied at Oxford University, Lives in London. Passionate about literature and currently working as an editor for a publishing company.
An abstract class is still a class, just like any other. Only interfaces can extends other interfaces. You can actually extend interfaces in Java, but it would still be called an interface. Then you can use this extended interface implemented in your abstract class.Aug 9, 2014
2023-06-11 06:42:26
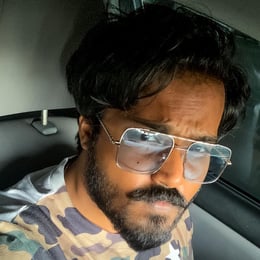
Benjamin Davis
QuesHub.com delivers expert answers and knowledge to you.
An abstract class is still a class, just like any other. Only interfaces can extends other interfaces. You can actually extend interfaces in Java, but it would still be called an interface. Then you can use this extended interface implemented in your abstract class.Aug 9, 2014