Is it possible to create object of interface in Java 2024?
I'll answer
Earn 20 gold coins for an accepted answer.20
Earn 20 gold coins for an accepted answer.
40more
40more
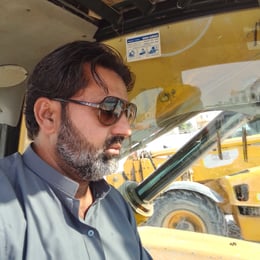
Felix Wilson
Works at the International Fund for Agricultural Development, Lives in Rome, Italy.
As a domain expert in Java, I'd like to clarify the concept of interfaces and their instantiation. In Java, an interface is a collection of abstract methods and, since Java 8, it can also include default methods and static methods. The primary purpose of an interface is to define a contract for classes to implement, ensuring that they provide specific behaviors.
Interfaces in Java are not meant to be instantiated directly. This is because they are essentially blueprints for classes. They define what methods a class should have but do not provide the implementation for those methods. An interface is a set of method signatures that a class can implement, and it's up to the class to provide the actual code for these methods.
The statement that "we cannot create an object of an interface" is correct. In Java, you cannot instantiate an interface directly because it does not have a constructor. Instead, you can create objects of classes that implement the interface. These classes provide the concrete implementation of the interface's methods.
However, with the introduction of default methods in Java 8, interfaces can now have a method with a default implementation. This allows interfaces to evolve without breaking existing implementations. Default methods are a way for interfaces to provide a default behavior for the methods they declare. This can be particularly useful when you want to add new functionality to an interface without forcing all implementing classes to reimplement the method.
Despite the ability to have default methods, interfaces still cannot be instantiated. The default methods serve as a way to provide a default implementation that can be used by any class that implements the interface, but they do not change the fact that an interface is an abstract type.
To utilize an interface, you would typically define it with the methods you want, and then create a class that implements the interface. Here's a simple example:
```java
public interface Vehicle {
void start();
void stop();
}
public class Car implements Vehicle {
@Override
public void start() {
System.out.println("Car is starting");
}
@Override
public void stop() {
System.out.println("Car is stopping");
}
}
// You can now create an object of Car and use it.
Car myCar = new Car();
myCar.start();
myCar.stop();
```
In this example, `Vehicle` is an interface with two abstract methods, `start` and `stop`. The `Car` class implements this interface and provides the concrete implementations for these methods. You can then create an instance of `Car` and call its methods.
To summarize, while interfaces play a crucial role in defining a contract for classes, they are not instantiated on their own. Instead, classes implement interfaces and provide the necessary implementations for the interface's methods. Default methods in interfaces offer a way to provide default implementations, but they do not change the fundamental nature of interfaces as abstract types.
Interfaces in Java are not meant to be instantiated directly. This is because they are essentially blueprints for classes. They define what methods a class should have but do not provide the implementation for those methods. An interface is a set of method signatures that a class can implement, and it's up to the class to provide the actual code for these methods.
The statement that "we cannot create an object of an interface" is correct. In Java, you cannot instantiate an interface directly because it does not have a constructor. Instead, you can create objects of classes that implement the interface. These classes provide the concrete implementation of the interface's methods.
However, with the introduction of default methods in Java 8, interfaces can now have a method with a default implementation. This allows interfaces to evolve without breaking existing implementations. Default methods are a way for interfaces to provide a default behavior for the methods they declare. This can be particularly useful when you want to add new functionality to an interface without forcing all implementing classes to reimplement the method.
Despite the ability to have default methods, interfaces still cannot be instantiated. The default methods serve as a way to provide a default implementation that can be used by any class that implements the interface, but they do not change the fact that an interface is an abstract type.
To utilize an interface, you would typically define it with the methods you want, and then create a class that implements the interface. Here's a simple example:
```java
public interface Vehicle {
void start();
void stop();
}
public class Car implements Vehicle {
@Override
public void start() {
System.out.println("Car is starting");
}
@Override
public void stop() {
System.out.println("Car is stopping");
}
}
// You can now create an object of Car and use it.
Car myCar = new Car();
myCar.start();
myCar.stop();
```
In this example, `Vehicle` is an interface with two abstract methods, `start` and `stop`. The `Car` class implements this interface and provides the concrete implementations for these methods. You can then create an instance of `Car` and call its methods.
To summarize, while interfaces play a crucial role in defining a contract for classes, they are not instantiated on their own. Instead, classes implement interfaces and provide the necessary implementations for the interface's methods. Default methods in interfaces offer a way to provide default implementations, but they do not change the fundamental nature of interfaces as abstract types.
2024-06-11 00:56:32
reply(1)
Helpful(1122)
Helpful
Helpful(2)
Studied at the University of Melbourne, Lives in Melbourne, Australia.
Because interface contains only abstract methods and as abstract methods do not have a body (of implementation code), we cannot create an object. Suppose even if it is permitted, what is the use. Calling the abstract method with object does not yield any purpose as no output.
2023-06-15 06:34:37
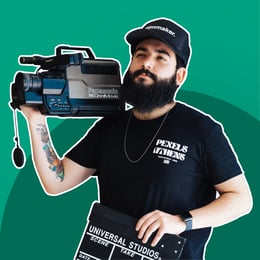
James Rodriguez
QuesHub.com delivers expert answers and knowledge to you.
Because interface contains only abstract methods and as abstract methods do not have a body (of implementation code), we cannot create an object. Suppose even if it is permitted, what is the use. Calling the abstract method with object does not yield any purpose as no output.