Can we extend an interface in Java?
I'll answer
Earn 20 gold coins for an accepted answer.20
Earn 20 gold coins for an accepted answer.
40more
40more
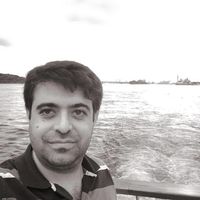
Ethan Davis
Works at the International Committee of the Red Cross, Lives in Geneva, Switzerland.
Hello! I'm an expert in Java programming, and I'm here to help you with your question about extending interfaces in Java.
In Java, a class can only extend one parent class, which means that single inheritance is the rule. However, Java does allow a class to implement multiple interfaces. This is a form of multiple inheritance, but it's done through interfaces rather than classes. When it comes to interfaces, they can extend other interfaces, and this is where the concept of interface extension comes into play.
The `extends` keyword is used when a class extends another class or when an interface extends another interface. When extending interfaces, the `extends` keyword is used once, followed by a comma-separated list of the parent interfaces that the new interface is extending. This allows for the creation of a hierarchy of interfaces, where a new interface can inherit the constants and abstract methods from its parent interfaces.
Here's an example to illustrate how interfaces can be extended in Java:
```java
public interface Animal {
void eat();
}
public interface Flyable {
void fly();
}
public interface Bat extends Animal, Flyable {
void echolocate();
}
```
In this example, the `Bat` interface extends both the `Animal` and `Flyable` interfaces. This means that any class that implements the `Bat` interface must provide implementations for the `eat()` and `fly()` methods inherited from the parent interfaces, as well as the `echolocate()` method that is specific to the `Bat` interface.
Interface extension in Java is a powerful feature that allows for the creation of flexible and reusable code. It enables developers to define common behavior in a base interface and then extend that behavior in more specific interfaces. This promotes code reuse and makes it easier to create a well-structured and maintainable codebase.
One important thing to note is that interfaces in Java can only contain abstract methods and final variables (constants). This means that the methods in an interface cannot have an implementation, and any variables must be declared as final and static. When a class implements an interface, it must provide an implementation for all of the abstract methods in the interface.
In summary, extending interfaces in Java is a key part of the language's support for multiple inheritance through interfaces. It allows developers to create a hierarchy of interfaces and define common behavior that can be reused across different parts of a program. By using interfaces effectively, you can write more flexible and maintainable code in Java.
In Java, a class can only extend one parent class, which means that single inheritance is the rule. However, Java does allow a class to implement multiple interfaces. This is a form of multiple inheritance, but it's done through interfaces rather than classes. When it comes to interfaces, they can extend other interfaces, and this is where the concept of interface extension comes into play.
The `extends` keyword is used when a class extends another class or when an interface extends another interface. When extending interfaces, the `extends` keyword is used once, followed by a comma-separated list of the parent interfaces that the new interface is extending. This allows for the creation of a hierarchy of interfaces, where a new interface can inherit the constants and abstract methods from its parent interfaces.
Here's an example to illustrate how interfaces can be extended in Java:
```java
public interface Animal {
void eat();
}
public interface Flyable {
void fly();
}
public interface Bat extends Animal, Flyable {
void echolocate();
}
```
In this example, the `Bat` interface extends both the `Animal` and `Flyable` interfaces. This means that any class that implements the `Bat` interface must provide implementations for the `eat()` and `fly()` methods inherited from the parent interfaces, as well as the `echolocate()` method that is specific to the `Bat` interface.
Interface extension in Java is a powerful feature that allows for the creation of flexible and reusable code. It enables developers to define common behavior in a base interface and then extend that behavior in more specific interfaces. This promotes code reuse and makes it easier to create a well-structured and maintainable codebase.
One important thing to note is that interfaces in Java can only contain abstract methods and final variables (constants). This means that the methods in an interface cannot have an implementation, and any variables must be declared as final and static. When a class implements an interface, it must provide an implementation for all of the abstract methods in the interface.
In summary, extending interfaces in Java is a key part of the language's support for multiple inheritance through interfaces. It allows developers to create a hierarchy of interfaces and define common behavior that can be reused across different parts of a program. By using interfaces effectively, you can write more flexible and maintainable code in Java.
2024-05-12 21:16:00
reply(1)
Helpful(1122)
Helpful
Helpful(2)
Works at BioHealth Labs, Lives in Boston, MA.
A Java class can only extend one parent class. Multiple inheritance is not allowed. Interfaces are not classes, however, and an interface can extend more than one parent interface. The extends keyword is used once, and the parent interfaces are declared in a comma-separated list.
2023-06-09 06:34:32
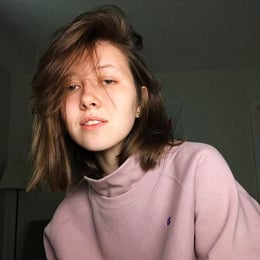
Lily Gonzales
QuesHub.com delivers expert answers and knowledge to you.
A Java class can only extend one parent class. Multiple inheritance is not allowed. Interfaces are not classes, however, and an interface can extend more than one parent interface. The extends keyword is used once, and the parent interfaces are declared in a comma-separated list.