Why do we need private constructor in Java?
I'll answer
Earn 20 gold coins for an accepted answer.20
Earn 20 gold coins for an accepted answer.
40more
40more

Oliver Thompson
Works at the International Renewable Energy Agency, Lives in Abu Dhabi, UAE.
Hello, I'm an expert in Java programming with a focus on design patterns and object-oriented principles. Let's delve into the reasons why a private constructor is used in Java, particularly in the context of the Singleton pattern.
Step 1: English Explanation
In Java, a private constructor is a class constructor that is not accessible outside the class in which it is declared. This means that it cannot be used to create new instances of the class from outside the class. The use of a private constructor is a common practice in Java for various reasons, and one of the most notable is its role in implementing the Singleton pattern.
### Singleton Pattern
The Singleton pattern is a software design pattern that ensures a class has only one instance and provides a global point of access to that instance. This is particularly useful when exactly one object is needed to coordinate actions across the system. For example, a singleton might be used for a configuration manager, a connection pool, or a thread-local variable holder.
### Why Private Constructor?
Here are several reasons why a private constructor is essential for the Singleton pattern:
1. Control Over Instance Creation: By making the constructor private, you prevent other classes from using the `new` keyword to create multiple instances of the Singleton class.
2. Lazy Initialization: A private constructor allows for lazy initialization, which means the Singleton instance is not created until it is needed. This can be beneficial for performance, especially if the instance is resource-intensive or expensive to create.
3. Thread Safety: When combined with synchronization techniques, a private constructor can help ensure that only one thread can create the Singleton instance at a time, preventing race conditions.
4. Global Access Point: A private constructor is often paired with a static method or a static variable that provides a global access point to the Singleton instance. This way, the Singleton can be accessed from anywhere in the application without the need to pass it around.
5. Enforcing Singleton: It enforces the Singleton property by not allowing other classes to subclass the Singleton class and create additional instances.
6. Design Integrity: It maintains the integrity of the design by ensuring that the Singleton class is not instantiated in ways that could violate its contract.
7.
Testability: Private constructors can improve the testability of your code by allowing you to mock or stub out the Singleton for testing purposes.
8.
Versioning and Maintenance: They can simplify versioning and maintenance by encapsulating the instantiation logic within the class itself, making it easier to change how the Singleton is created in the future without affecting external code.
### Example of Singleton with Private Constructor
Here's a simple example of how a private constructor is used in a Singleton class:
```java
public class Singleton {
// The private static variable that holds the single instance
private static Singleton instance;
// Private constructor so no other class can instantiate it
private Singleton() {
// Initialization code
}
// Public static method that returns the instance of the class
public static Singleton getInstance() {
if (instance == null) {
// Synchronized block to make the method thread-safe
synchronized (Singleton.class) {
// Double-check locking pattern
if (instance == null) {
instance = new Singleton();
}
}
}
return instance;
}
// Other methods
}
```
In this example, the `Singleton` class has a private constructor, which is only accessible within the class itself. The `getInstance` method provides a controlled way to access the Singleton instance, ensuring that only one instance is created.
Step 2: Divider
Step 1: English Explanation
In Java, a private constructor is a class constructor that is not accessible outside the class in which it is declared. This means that it cannot be used to create new instances of the class from outside the class. The use of a private constructor is a common practice in Java for various reasons, and one of the most notable is its role in implementing the Singleton pattern.
### Singleton Pattern
The Singleton pattern is a software design pattern that ensures a class has only one instance and provides a global point of access to that instance. This is particularly useful when exactly one object is needed to coordinate actions across the system. For example, a singleton might be used for a configuration manager, a connection pool, or a thread-local variable holder.
### Why Private Constructor?
Here are several reasons why a private constructor is essential for the Singleton pattern:
1. Control Over Instance Creation: By making the constructor private, you prevent other classes from using the `new` keyword to create multiple instances of the Singleton class.
2. Lazy Initialization: A private constructor allows for lazy initialization, which means the Singleton instance is not created until it is needed. This can be beneficial for performance, especially if the instance is resource-intensive or expensive to create.
3. Thread Safety: When combined with synchronization techniques, a private constructor can help ensure that only one thread can create the Singleton instance at a time, preventing race conditions.
4. Global Access Point: A private constructor is often paired with a static method or a static variable that provides a global access point to the Singleton instance. This way, the Singleton can be accessed from anywhere in the application without the need to pass it around.
5. Enforcing Singleton: It enforces the Singleton property by not allowing other classes to subclass the Singleton class and create additional instances.
6. Design Integrity: It maintains the integrity of the design by ensuring that the Singleton class is not instantiated in ways that could violate its contract.
7.
Testability: Private constructors can improve the testability of your code by allowing you to mock or stub out the Singleton for testing purposes.
8.
Versioning and Maintenance: They can simplify versioning and maintenance by encapsulating the instantiation logic within the class itself, making it easier to change how the Singleton is created in the future without affecting external code.
### Example of Singleton with Private Constructor
Here's a simple example of how a private constructor is used in a Singleton class:
```java
public class Singleton {
// The private static variable that holds the single instance
private static Singleton instance;
// Private constructor so no other class can instantiate it
private Singleton() {
// Initialization code
}
// Public static method that returns the instance of the class
public static Singleton getInstance() {
if (instance == null) {
// Synchronized block to make the method thread-safe
synchronized (Singleton.class) {
// Double-check locking pattern
if (instance == null) {
instance = new Singleton();
}
}
}
return instance;
}
// Other methods
}
```
In this example, the `Singleton` class has a private constructor, which is only accessible within the class itself. The `getInstance` method provides a controlled way to access the Singleton instance, ensuring that only one instance is created.
Step 2: Divider
2024-05-12 21:15:18
reply(1)
Helpful(1122)
Helpful
Helpful(2)
Works at the United Nations Office on Drugs and Crime, Lives in Vienna, Austria.
Java -C private constructor example. The use of private constructor is to serve singleton classes. A singleton class is one which limits the number of objects creation to one. Using private constructor we can ensure that no more than one object can be created at a time.
2023-06-19 06:34:32
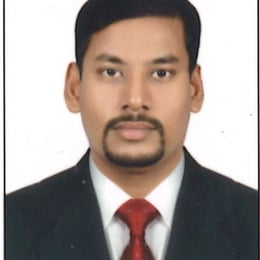
Benjamin Stewart
QuesHub.com delivers expert answers and knowledge to you.
Java -C private constructor example. The use of private constructor is to serve singleton classes. A singleton class is one which limits the number of objects creation to one. Using private constructor we can ensure that no more than one object can be created at a time.