What are the types of polymorphism in Java?
I'll answer
Earn 20 gold coins for an accepted answer.20
Earn 20 gold coins for an accepted answer.
40more
40more

Emily Turner
Studied at Stanford University, Lives in Palo Alto. Currently working as a software engineer for a tech startup.
As an expert in the field of computer science with a focus on Java programming, I'd like to provide a comprehensive explanation of polymorphism in Java. Polymorphism is a fundamental concept in object-oriented programming (OOP) that allows objects to be treated as instances of their parent class rather than their actual class. This enables a single interface to be used for a general class of actions. In Java, polymorphism can be categorized into two main types: compile-time polymorphism and runtime polymorphism.
**Compile-time Polymorphism (Static Binding)**:
Compile-time polymorphism, also known as static polymorphism or method overloading, occurs when multiple methods have the same name but differ in the number and/or type of parameters. The compiler determines which method to invoke based on the method signature, which includes the method name and the parameter list. Since the decision is made at compile time, it is also referred to as static binding.
Method overloading is a common example of compile-time polymorphism. Here, a class can have multiple methods with the same name but with different parameter lists. The correct method to call is determined at compile time based on the arguments used when the method is invoked.
Runtime Polymorphism (Dynamic Binding):
Runtime polymorphism, also known as dynamic polymorphism or method overriding, occurs when a subclass provides a specific implementation of a method that is already defined in its superclass. The method name and the parameter list remain the same, but the implementation differs. The decision about which method to invoke is made at runtime, which is why it is called dynamic binding.
Method overriding is a key example of runtime polymorphism. When a subclass overrides a method of a superclass, it provides its own implementation of that method. The call to the method is resolved at runtime based on the object that invokes the method, not the reference type.
Advantages of Polymorphism:
1. Code Reusability: Polymorphism allows the same code to work with different data types.
2. Loose Coupling: It promotes loose coupling between software components, making the system more modular and flexible.
3. Maintainability: Code is easier to maintain and extend due to its modular nature.
4. Interchangeability: Objects of different classes can be interchangeable if they are subclasses of the same parent class.
Example of Polymorphism in Java:
```java
class Animal {
public void makeSound() {
System.out.println("Some sound");
}
}
class Dog extends Animal {
@Override
public void makeSound() {
System.out.println("Woof woof");
}
}
class Cat extends Animal {
@Override
public void makeSound() {
System.out.println("Meow");
}
}
public class Main {
public static void main(String[] args) {
Animal myDog = new Dog();
Animal myCat = new Cat();
// Compile-time polymorphism (method overloading not shown)
// Runtime polymorphism (method overriding)
myDog.makeSound(); // Outputs: Woof woof
myCat.makeSound(); // Outputs: Meow
}
}
```
In this example, `Animal` is a superclass with a method `makeSound()`. The classes `Dog` and `Cat` are subclasses that override the `makeSound()` method to provide their own implementation. When we call `makeSound()` using instances of `Dog` and `Cat`, the appropriate method is called based on the actual object type, not the reference type, demonstrating runtime polymorphism.
Polymorphism is a powerful feature of Java that enhances the language's expressiveness and flexibility, allowing developers to write more generic and reusable code.
**Compile-time Polymorphism (Static Binding)**:
Compile-time polymorphism, also known as static polymorphism or method overloading, occurs when multiple methods have the same name but differ in the number and/or type of parameters. The compiler determines which method to invoke based on the method signature, which includes the method name and the parameter list. Since the decision is made at compile time, it is also referred to as static binding.
Method overloading is a common example of compile-time polymorphism. Here, a class can have multiple methods with the same name but with different parameter lists. The correct method to call is determined at compile time based on the arguments used when the method is invoked.
Runtime Polymorphism (Dynamic Binding):
Runtime polymorphism, also known as dynamic polymorphism or method overriding, occurs when a subclass provides a specific implementation of a method that is already defined in its superclass. The method name and the parameter list remain the same, but the implementation differs. The decision about which method to invoke is made at runtime, which is why it is called dynamic binding.
Method overriding is a key example of runtime polymorphism. When a subclass overrides a method of a superclass, it provides its own implementation of that method. The call to the method is resolved at runtime based on the object that invokes the method, not the reference type.
Advantages of Polymorphism:
1. Code Reusability: Polymorphism allows the same code to work with different data types.
2. Loose Coupling: It promotes loose coupling between software components, making the system more modular and flexible.
3. Maintainability: Code is easier to maintain and extend due to its modular nature.
4. Interchangeability: Objects of different classes can be interchangeable if they are subclasses of the same parent class.
Example of Polymorphism in Java:
```java
class Animal {
public void makeSound() {
System.out.println("Some sound");
}
}
class Dog extends Animal {
@Override
public void makeSound() {
System.out.println("Woof woof");
}
}
class Cat extends Animal {
@Override
public void makeSound() {
System.out.println("Meow");
}
}
public class Main {
public static void main(String[] args) {
Animal myDog = new Dog();
Animal myCat = new Cat();
// Compile-time polymorphism (method overloading not shown)
// Runtime polymorphism (method overriding)
myDog.makeSound(); // Outputs: Woof woof
myCat.makeSound(); // Outputs: Meow
}
}
```
In this example, `Animal` is a superclass with a method `makeSound()`. The classes `Dog` and `Cat` are subclasses that override the `makeSound()` method to provide their own implementation. When we call `makeSound()` using instances of `Dog` and `Cat`, the appropriate method is called based on the actual object type, not the reference type, demonstrating runtime polymorphism.
Polymorphism is a powerful feature of Java that enhances the language's expressiveness and flexibility, allowing developers to write more generic and reusable code.
2024-05-12 21:10:49
reply(1)
Helpful(1122)
Helpful
Helpful(2)
Studied at the University of Sydney, Lives in Sydney, Australia.
Polymorphism in Java has two types: Compile time polymorphism (static binding) and Runtime polymorphism (dynamic binding). Method overloading is an example of static polymorphism, while method overriding is an example of dynamic polymorphism.Jan 15, 2013
2023-06-15 06:34:31
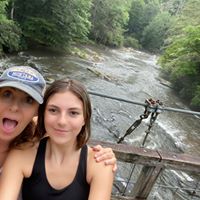
Charlotte Clark
QuesHub.com delivers expert answers and knowledge to you.
Polymorphism in Java has two types: Compile time polymorphism (static binding) and Runtime polymorphism (dynamic binding). Method overloading is an example of static polymorphism, while method overriding is an example of dynamic polymorphism.Jan 15, 2013