What is the difference between inheritance and polymorphism?
I'll answer
Earn 20 gold coins for an accepted answer.20
Earn 20 gold coins for an accepted answer.
40more
40more
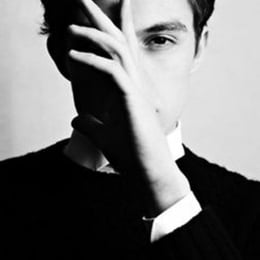
Daniel Wright
Works at Microsoft, Lives in Seattle. Graduated from University of Washington with a degree in Computer Science.
In the realm of object-oriented programming (OOP), inheritance and polymorphism are two fundamental concepts that are often discussed in tandem. However, they serve different purposes and operate on distinct principles within the OOP paradigm. Let's delve into the nuances of each concept and explore the differences between them.
Inheritance is a mechanism in OOP where a new class (known as the subclass or derived class) is created from an existing class (known as the superclass or base class). The subclass inherits attributes and behaviors from the superclass and can introduce new ones or override the existing ones. It is a way to achieve code reuse and create a hierarchy of classes that can share common characteristics and functionalities.
Inheritance allows for the creation of a more specific class from a general one. For instance, if you have a `Vehicle` class with properties like `wheels` and methods like `drive()`, you can create more specific classes like `Car`, `Bicycle`, and `Motorcycle` that inherit from `Vehicle`. Each of these subclasses can have additional properties and methods specific to them, such as `Car` having a `trunkSize` property or `Motorcycle` having a different implementation of the `drive()` method.
Polymorphism, on the other hand, is the ability of an object to take on many forms. In OOP, polymorphism allows methods to do different things based on the actual object that is invoking them. It is a feature that enables a single interface to be used for a general class of actions. There are two primary types of polymorphism: compile-time (also known as static polymorphism) and runtime (also known as dynamic polymorphism).
Compile-time polymorphism is achieved through method overloading and operator overloading, where the compiler determines which method to invoke based on the method signature (the number and type of parameters).
Runtime polymorphism is achieved through method overriding, where a subclass provides a specific implementation of a method that is already provided by its superclass. The decision about which method to invoke is made at runtime based on the type of the object that is calling the method.
Here's a key distinction: inheritance is a design principle that allows for the creation of new classes based on existing ones, promoting code reuse and establishing a relationship between classes.
Polymorphism is a behavior that allows objects to be treated as instances of their parent class rather than their actual class. This behavior is particularly useful when you want to write code that can work with objects of different types through a uniform interface.
To illustrate the difference with an example, consider a class hierarchy where `Bird` is a subclass of `Animal`. Inheritance allows a `Bird` to inherit properties and methods from `Animal`, such as `eat()` and `sleep()`. Polymorphism comes into play when you have a method that works with `Animal` objects and you want it to behave differently when it's a `Bird`. For example, an `Animal` might `walk()`, but a `Bird` might `fly()`. When you invoke the `move()` method on an `Animal` object, it will `walk()`, but if you invoke it on a `Bird` object, it will `fly()`, even though both are treated as `Animal` objects due to polymorphism.
In summary, inheritance is about creating a new class from an existing class, allowing for a hierarchical organization of classes, while polymorphism is about allowing a single interface to represent different types of operations. Inheritance is a structural concept, whereas polymorphism is a behavioral concept.
Now, let's proceed with the translation into Chinese.
Inheritance is a mechanism in OOP where a new class (known as the subclass or derived class) is created from an existing class (known as the superclass or base class). The subclass inherits attributes and behaviors from the superclass and can introduce new ones or override the existing ones. It is a way to achieve code reuse and create a hierarchy of classes that can share common characteristics and functionalities.
Inheritance allows for the creation of a more specific class from a general one. For instance, if you have a `Vehicle` class with properties like `wheels` and methods like `drive()`, you can create more specific classes like `Car`, `Bicycle`, and `Motorcycle` that inherit from `Vehicle`. Each of these subclasses can have additional properties and methods specific to them, such as `Car` having a `trunkSize` property or `Motorcycle` having a different implementation of the `drive()` method.
Polymorphism, on the other hand, is the ability of an object to take on many forms. In OOP, polymorphism allows methods to do different things based on the actual object that is invoking them. It is a feature that enables a single interface to be used for a general class of actions. There are two primary types of polymorphism: compile-time (also known as static polymorphism) and runtime (also known as dynamic polymorphism).
Compile-time polymorphism is achieved through method overloading and operator overloading, where the compiler determines which method to invoke based on the method signature (the number and type of parameters).
Runtime polymorphism is achieved through method overriding, where a subclass provides a specific implementation of a method that is already provided by its superclass. The decision about which method to invoke is made at runtime based on the type of the object that is calling the method.
Here's a key distinction: inheritance is a design principle that allows for the creation of new classes based on existing ones, promoting code reuse and establishing a relationship between classes.
Polymorphism is a behavior that allows objects to be treated as instances of their parent class rather than their actual class. This behavior is particularly useful when you want to write code that can work with objects of different types through a uniform interface.
To illustrate the difference with an example, consider a class hierarchy where `Bird` is a subclass of `Animal`. Inheritance allows a `Bird` to inherit properties and methods from `Animal`, such as `eat()` and `sleep()`. Polymorphism comes into play when you have a method that works with `Animal` objects and you want it to behave differently when it's a `Bird`. For example, an `Animal` might `walk()`, but a `Bird` might `fly()`. When you invoke the `move()` method on an `Animal` object, it will `walk()`, but if you invoke it on a `Bird` object, it will `fly()`, even though both are treated as `Animal` objects due to polymorphism.
In summary, inheritance is about creating a new class from an existing class, allowing for a hierarchical organization of classes, while polymorphism is about allowing a single interface to represent different types of operations. Inheritance is a structural concept, whereas polymorphism is a behavioral concept.
Now, let's proceed with the translation into Chinese.
2024-05-12 21:10:38
reply(1)
Helpful(1122)
Helpful
Helpful(2)
Works at Digital Marketing Agency, Lives in Dublin, Ireland.
The main difference is polymorphism is a specific result of inheritance. Polymorphism is where the method to be invoked is determined at runtime based on the type of the object. This is a situation that results when you have one class inheriting from another and overriding a particular method.Jun 10, 2011
2023-06-13 06:34:30
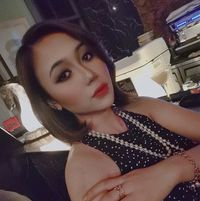
Amelia Patel
QuesHub.com delivers expert answers and knowledge to you.
The main difference is polymorphism is a specific result of inheritance. Polymorphism is where the method to be invoked is determined at runtime based on the type of the object. This is a situation that results when you have one class inheriting from another and overriding a particular method.Jun 10, 2011