Why should we use polymorphism?
I'll answer
Earn 20 gold coins for an accepted answer.20
Earn 20 gold coins for an accepted answer.
40more
40more

Lucas Phillips
Works at the International Development Association, Lives in Washington, D.C., USA.
As a domain expert in software engineering with a focus on object-oriented programming (OOP), I'd like to elucidate the significance of polymorphism in the context of Java and other OOP languages. Polymorphism is a fundamental concept that allows for the use of a single interface to represent different underlying forms (data types). Here's why it's crucial:
### Flexibility and Reusability
Polymorphism allows developers to write code that can work with objects of different types and classes. This means that a single piece of code can be reused with different objects, as long as they adhere to the same interface. This enhances the flexibility of the code and reduces redundancy.
### Abstraction
It enables a higher level of abstraction. By focusing on the common interface rather than the specific implementation details, developers can create more abstract and generic code. This abstraction simplifies the design and maintenance of complex systems.
### Extensibility
Polymorphism supports the open/closed principle, which states that software entities should be open for extension but closed for modification. New classes can be introduced without altering the existing code that uses polymorphic behavior, thus making the system more extensible.
### Decoupling
It helps in decoupling the code by allowing the definition and implementation to be separated. This means that the code that uses polymorphism doesn't need to know the specifics of how a method is implemented, as long as it adheres to the interface.
### Maintainability
Code that uses polymorphism tends to be more maintainable. Since the implementation details are hidden behind an interface, changes to the underlying implementation do not affect the code that uses the interface.
### Testability
It improves the testability of the code. Since polymorphism allows for the use of different implementations through a common interface, it becomes easier to mock objects for testing purposes.
### Interchangeability
Objects of different classes can be interchangeable if they follow the same interface. This means that an object can be replaced with another object of a different class as long as the new object can perform the same tasks defined by the interface.
### Example in Java
In Java, polymorphism is often implemented through inheritance and interfaces. When a parent class reference is used to refer to a child class object, the child class can override the parent class methods to provide specific behavior. This allows for a common way to handle different classes of objects.
For instance, consider a `Shape` class with a method `draw()`. There could be various subclasses like `Circle`, `Square`, and `Triangle`, each providing their own implementation of the `draw()` method. Through polymorphism, you can pass any of these objects to a function that expects a `Shape`, and the correct `draw()` method will be called based on the actual object's type at runtime.
### Conclusion
Polymorphism is a powerful concept that enhances the capabilities of OOP languages like Java. It allows for more flexible, abstract, and maintainable code. By leveraging polymorphism, developers can create systems that are easier to extend and modify, which ultimately leads to more robust and scalable software solutions.
### Flexibility and Reusability
Polymorphism allows developers to write code that can work with objects of different types and classes. This means that a single piece of code can be reused with different objects, as long as they adhere to the same interface. This enhances the flexibility of the code and reduces redundancy.
### Abstraction
It enables a higher level of abstraction. By focusing on the common interface rather than the specific implementation details, developers can create more abstract and generic code. This abstraction simplifies the design and maintenance of complex systems.
### Extensibility
Polymorphism supports the open/closed principle, which states that software entities should be open for extension but closed for modification. New classes can be introduced without altering the existing code that uses polymorphic behavior, thus making the system more extensible.
### Decoupling
It helps in decoupling the code by allowing the definition and implementation to be separated. This means that the code that uses polymorphism doesn't need to know the specifics of how a method is implemented, as long as it adheres to the interface.
### Maintainability
Code that uses polymorphism tends to be more maintainable. Since the implementation details are hidden behind an interface, changes to the underlying implementation do not affect the code that uses the interface.
### Testability
It improves the testability of the code. Since polymorphism allows for the use of different implementations through a common interface, it becomes easier to mock objects for testing purposes.
### Interchangeability
Objects of different classes can be interchangeable if they follow the same interface. This means that an object can be replaced with another object of a different class as long as the new object can perform the same tasks defined by the interface.
### Example in Java
In Java, polymorphism is often implemented through inheritance and interfaces. When a parent class reference is used to refer to a child class object, the child class can override the parent class methods to provide specific behavior. This allows for a common way to handle different classes of objects.
For instance, consider a `Shape` class with a method `draw()`. There could be various subclasses like `Circle`, `Square`, and `Triangle`, each providing their own implementation of the `draw()` method. Through polymorphism, you can pass any of these objects to a function that expects a `Shape`, and the correct `draw()` method will be called based on the actual object's type at runtime.
### Conclusion
Polymorphism is a powerful concept that enhances the capabilities of OOP languages like Java. It allows for more flexible, abstract, and maintainable code. By leveraging polymorphism, developers can create systems that are easier to extend and modify, which ultimately leads to more robust and scalable software solutions.
2024-05-12 12:15:37
reply(1)
Helpful(1122)
Helpful
Helpful(2)
Studied at Yale University, Lives in New Haven, CT
The good reason for why Polymorphism is need in java is because the concept is extensively used in implementing inheritance.it plays an important role in allowing objects having different internal structures to share the same external interface.
2023-06-14 06:34:27
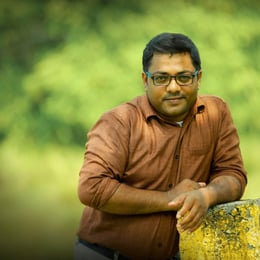
Ethan Moore
QuesHub.com delivers expert answers and knowledge to you.
The good reason for why Polymorphism is need in java is because the concept is extensively used in implementing inheritance.it plays an important role in allowing objects having different internal structures to share the same external interface.