Why we are not able to create object of interface?
I'll answer
Earn 20 gold coins for an accepted answer.20
Earn 20 gold coins for an accepted answer.
40more
40more

Julian Wilson
Works at the International Seabed Authority, Lives in Kingston, Jamaica.
Hello, I'm a software development expert with a strong focus on object-oriented programming and design principles. I'm here to help you understand why we can't create an object of an interface in Java and other similar programming languages.
Interfaces are a fundamental concept in object-oriented programming, providing a way to define a contract for a set of methods that a class can implement. They are essentially a blueprint for what a class can do, without specifying how it should do it. This allows for a high degree of flexibility and abstraction in your code.
Here are a few key points to consider:
1. Abstraction: Interfaces are purely abstract. They define methods without providing any implementation. This means that they are a set of promises about what a class can do, but not how it will do it.
2. Multiple Inheritance: In Java, a class can only inherit from one other class (single inheritance), but it can implement multiple interfaces. This allows a class to inherit behavior from multiple sources.
3. Compatibility: Interfaces allow for runtime polymorphism. When you have a reference to an interface, you can be sure that any object assigned to that reference will have the methods defined in that interface.
4. Design by Contract: Interfaces enforce a design by contract principle. When a class implements an interface, it promises to provide an implementation for all the methods declared in that interface.
Now, let's address the question of why we can't create an object of an interface:
Interfaces are designed to be implemented, not instantiated. When you define an interface, you're creating a type that specifies a set of methods that a class must implement. Since interfaces contain no implementation details, there's nothing to instantiate. If you were to try to create an object of an interface directly, you would get a compilation error because there is no code to compile for the methods defined in the interface.
Here's a simple example in Java to illustrate:
```java
interface Vehicle {
void drive();
}
public class Car implements Vehicle {
public void drive() {
System.out.println("The car is driving.");
}
}
public class Main {
public static void main(String[] args) {
// This will not compile because Vehicle is an interface and cannot be instantiated
// Vehicle myVehicle = new Vehicle();
// This will compile and run because Car is a class that implements the Vehicle interface
Vehicle myVehicle = new Car();
myVehicle.drive(); // Outputs: The car is driving.
}
}
```
In the example above, `Vehicle` is an interface that specifies a `drive()` method. The `Car` class implements the `Vehicle` interface and provides an implementation for the `drive()` method. We can create an object of `Car` and assign it to a `Vehicle` reference because `Car` fulfills the contract specified by the `Vehicle` interface.
To summarize, interfaces are a powerful tool for defining capabilities and ensuring compatibility between different classes. They are not meant to be instantiated directly because they do not provide any implementation. Instead, they are implemented by classes, which can then be instantiated and used.
Interfaces are a fundamental concept in object-oriented programming, providing a way to define a contract for a set of methods that a class can implement. They are essentially a blueprint for what a class can do, without specifying how it should do it. This allows for a high degree of flexibility and abstraction in your code.
Here are a few key points to consider:
1. Abstraction: Interfaces are purely abstract. They define methods without providing any implementation. This means that they are a set of promises about what a class can do, but not how it will do it.
2. Multiple Inheritance: In Java, a class can only inherit from one other class (single inheritance), but it can implement multiple interfaces. This allows a class to inherit behavior from multiple sources.
3. Compatibility: Interfaces allow for runtime polymorphism. When you have a reference to an interface, you can be sure that any object assigned to that reference will have the methods defined in that interface.
4. Design by Contract: Interfaces enforce a design by contract principle. When a class implements an interface, it promises to provide an implementation for all the methods declared in that interface.
Now, let's address the question of why we can't create an object of an interface:
Interfaces are designed to be implemented, not instantiated. When you define an interface, you're creating a type that specifies a set of methods that a class must implement. Since interfaces contain no implementation details, there's nothing to instantiate. If you were to try to create an object of an interface directly, you would get a compilation error because there is no code to compile for the methods defined in the interface.
Here's a simple example in Java to illustrate:
```java
interface Vehicle {
void drive();
}
public class Car implements Vehicle {
public void drive() {
System.out.println("The car is driving.");
}
}
public class Main {
public static void main(String[] args) {
// This will not compile because Vehicle is an interface and cannot be instantiated
// Vehicle myVehicle = new Vehicle();
// This will compile and run because Car is a class that implements the Vehicle interface
Vehicle myVehicle = new Car();
myVehicle.drive(); // Outputs: The car is driving.
}
}
```
In the example above, `Vehicle` is an interface that specifies a `drive()` method. The `Car` class implements the `Vehicle` interface and provides an implementation for the `drive()` method. We can create an object of `Car` and assign it to a `Vehicle` reference because `Car` fulfills the contract specified by the `Vehicle` interface.
To summarize, interfaces are a powerful tool for defining capabilities and ensuring compatibility between different classes. They are not meant to be instantiated directly because they do not provide any implementation. Instead, they are implemented by classes, which can then be instantiated and used.
2024-05-12 12:15:48
reply(1)
Helpful(1122)
Helpful
Helpful(2)
Works at the International Seabed Authority, Lives in Kingston, Jamaica.
We can't create object of interfaces because of the reason that : Interface is basically a complete abstract class. ... So if we don't have any implementation of a method then that means if we create object of that interface and call that method it compile nothing as there is no code to compile.
2023-06-14 06:34:27
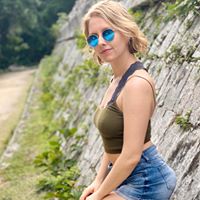
Charlotte Young
QuesHub.com delivers expert answers and knowledge to you.
We can't create object of interfaces because of the reason that : Interface is basically a complete abstract class. ... So if we don't have any implementation of a method then that means if we create object of that interface and call that method it compile nothing as there is no code to compile.