Why an interface is required in Java?
I'll answer
Earn 20 gold coins for an accepted answer.20
Earn 20 gold coins for an accepted answer.
40more
40more
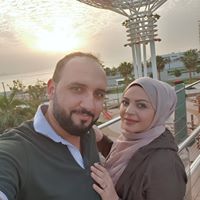
Ethan Walker
Works at the International Criminal Court, Lives in The Hague, Netherlands.
As a domain expert in Java programming, I can provide insights into the importance of interfaces in the language. Interfaces in Java are a fundamental concept that serve several key purposes and offer numerous benefits. Here's a detailed explanation:
1. Abstraction: Interfaces allow for the abstraction of behavior. They define a contract that any implementing class must adhere to. This means that the class agrees to implement all the methods defined by the interface, which can be crucial for ensuring that different classes can work together in a predictable way.
2. Multiple Inheritance: Java does not support multiple inheritance for classes. However, a class can implement multiple interfaces. This is a powerful feature because it allows a class to inherit behavior from multiple sources, thus providing the flexibility that multiple inheritance would offer.
3. Decoupling: Interfaces promote loose coupling between classes. By programming to an interface rather than a concrete implementation, you can write code that is not tightly bound to a specific class. This makes the code more maintainable and easier to change or extend.
4. Contract for Providers and Consumers: Interfaces serve as a contract between the providers and consumers of a service. Providers implement the interface, and consumers use the interface to interact with the service without needing to know the underlying implementation details.
5. Default Methods: Since Java 8, interfaces can contain default methods with an implementation. This allows interfaces to evolve over time without breaking existing implementations. Default methods can provide common default behavior that implementing classes can choose to inherit or override.
6. Marking Methods as Overridable: Interfaces can be used to mark certain methods as overridable, which can be useful when creating frameworks or libraries where you want to allow users to extend functionality in a controlled way.
7. Type Safety: Interfaces provide a level of type safety. When you declare a variable as an interface type, you are ensuring that it will only hold objects that implement that interface, regardless of the specific class that implements it.
8. Ease of Maintenance: When an interface changes, it is often easier to update the code because the changes are limited to the interface itself and the default methods it may contain. Implementing classes can remain unchanged if they are already compliant with the interface.
9. Design Patterns: Interfaces are a cornerstone of many design patterns such as Strategy, Observer, and Command. They enable the patterns to be implemented in a clean and decoupled manner.
10. Testing and Mocking: Interfaces facilitate easier unit testing and mocking. Since interfaces define a clear contract, it is straightforward to create mock objects that adhere to the interface for testing purposes.
In summary, interfaces are a critical component of Java's design and are essential for creating flexible, maintainable, and robust code. They provide a way to achieve polymorphism, enforce a contract, and allow for the implementation of design patterns that are fundamental to object-oriented programming.
Now, let's move on to the translation:
1. Abstraction: Interfaces allow for the abstraction of behavior. They define a contract that any implementing class must adhere to. This means that the class agrees to implement all the methods defined by the interface, which can be crucial for ensuring that different classes can work together in a predictable way.
2. Multiple Inheritance: Java does not support multiple inheritance for classes. However, a class can implement multiple interfaces. This is a powerful feature because it allows a class to inherit behavior from multiple sources, thus providing the flexibility that multiple inheritance would offer.
3. Decoupling: Interfaces promote loose coupling between classes. By programming to an interface rather than a concrete implementation, you can write code that is not tightly bound to a specific class. This makes the code more maintainable and easier to change or extend.
4. Contract for Providers and Consumers: Interfaces serve as a contract between the providers and consumers of a service. Providers implement the interface, and consumers use the interface to interact with the service without needing to know the underlying implementation details.
5. Default Methods: Since Java 8, interfaces can contain default methods with an implementation. This allows interfaces to evolve over time without breaking existing implementations. Default methods can provide common default behavior that implementing classes can choose to inherit or override.
6. Marking Methods as Overridable: Interfaces can be used to mark certain methods as overridable, which can be useful when creating frameworks or libraries where you want to allow users to extend functionality in a controlled way.
7. Type Safety: Interfaces provide a level of type safety. When you declare a variable as an interface type, you are ensuring that it will only hold objects that implement that interface, regardless of the specific class that implements it.
8. Ease of Maintenance: When an interface changes, it is often easier to update the code because the changes are limited to the interface itself and the default methods it may contain. Implementing classes can remain unchanged if they are already compliant with the interface.
9. Design Patterns: Interfaces are a cornerstone of many design patterns such as Strategy, Observer, and Command. They enable the patterns to be implemented in a clean and decoupled manner.
10. Testing and Mocking: Interfaces facilitate easier unit testing and mocking. Since interfaces define a clear contract, it is straightforward to create mock objects that adhere to the interface for testing purposes.
In summary, interfaces are a critical component of Java's design and are essential for creating flexible, maintainable, and robust code. They provide a way to achieve polymorphism, enforce a contract, and allow for the implementation of design patterns that are fundamental to object-oriented programming.
Now, let's move on to the translation:
2024-05-12 12:15:58
reply(1)
Helpful(1122)
Helpful
Helpful(2)
Works at EcoVenture Consulting, Lives in Sydney, Australia.
Interfaces are more flexible, because a class can implement multiple interfaces. Since Java does not have multiple inheritance, using abstract classes prevents your users from using any other class hierarchy. In general, prefer interfaces when there are no default implementations or state.
2023-06-11 06:34:26

Olivia Walker
QuesHub.com delivers expert answers and knowledge to you.
Interfaces are more flexible, because a class can implement multiple interfaces. Since Java does not have multiple inheritance, using abstract classes prevents your users from using any other class hierarchy. In general, prefer interfaces when there are no default implementations or state.