What is a class and an object?
I'll answer
Earn 20 gold coins for an accepted answer.20
Earn 20 gold coins for an accepted answer.
40more
40more

Matthew Gonzalez
Works at Netflix, Lives in Los Gatos, CA
Hi there, I'm a seasoned expert in the field of computer science with a focus on object-oriented programming (OOP). Let's dive into the concepts of classes and objects, which are fundamental to OOP.
### What is a Class?
In the realm of OOP, a class is essentially a blueprint or template for creating objects. It defines the structure and behavior that the objects of its type will have. This includes data members (also known as attributes or properties) and member functions (also known as methods). The class serves as a guide for creating specific instances, which are the objects.
#### Key Features of a Class:
1. Abstraction: Classes provide a way to encapsulate data and behavior, abstracting the complexity of the real world into a simpler, more manageable form.
2. Encapsulation: They bundle the data and methods that operate on the data within a single unit, protecting it from external interference and misuse.
3. Inheritance: Classes can inherit characteristics from other classes, allowing for the creation of a hierarchy of classes that can reuse and extend existing functionality.
4. Polymorphism: This is the ability for a child class to take on many forms, allowing it to be treated as instances of its parent class.
### What is an Object?
An object is an instance of a class. It is a specific realization of the general template provided by the class. When you create an object, you are creating a unique entity that has its own state (data) and behavior (methods).
#### Key Features of an Object:
1. State: Each object maintains its own state. For example, if you have a `Dog` class, each `Dog` object may have different values for its attributes like `color`, `name`, and `breed`.
2. Behavior: Objects can perform actions defined by their class. Continuing with the `Dog` example, behaviors could include `wagging the tail`, `barking`, and `eating`.
3. Identity: Each object has a unique identity, which distinguishes it from other objects, even if they are of the same class.
### The Relationship Between Classes and Objects:
- Creation: Classes are used to create objects. You can think of a class as a cookie cutter, and objects are the cookies it produces.
- Inheritance: Objects inherit properties and behaviors from their class. If a `Dog` class has a `bark` method, every `Dog` object will have access to that method.
- Interaction: Objects interact with each other through messages, which are essentially method calls.
### Example:
Let's consider a simple example to illustrate the concept further. Imagine a `Vehicle` class with attributes like `make`, `model`, and `year`, and behaviors like `start`, `stop`, and `honk`.
```java
public class Vehicle {
String make;
String model;
int year;
public void start() {
// Code to start the vehicle
}
public void stop() {
// Code to stop the vehicle
}
public void honk() {
// Code to make the vehicle honk
}
}
```
Now, if you create an object from this class:
```java
Vehicle myCar = new Vehicle();
myCar.make = "Toyota";
myCar.model = "Corolla";
myCar.year = 2020;
```
Here, `myCar` is an instance of the `Vehicle` class. It has its own state (make, model, year) and can perform the behaviors defined in the class (start, stop, honk).
### Conclusion:
Understanding the distinction between classes and objects is crucial for grasping the principles of OOP. Classes are the blueprints that define the structure and behavior of objects, while objects are the tangible entities that are created from these blueprints. They encapsulate data and functionality, allowing for the creation of complex systems that are easier to manage and extend.
### What is a Class?
In the realm of OOP, a class is essentially a blueprint or template for creating objects. It defines the structure and behavior that the objects of its type will have. This includes data members (also known as attributes or properties) and member functions (also known as methods). The class serves as a guide for creating specific instances, which are the objects.
#### Key Features of a Class:
1. Abstraction: Classes provide a way to encapsulate data and behavior, abstracting the complexity of the real world into a simpler, more manageable form.
2. Encapsulation: They bundle the data and methods that operate on the data within a single unit, protecting it from external interference and misuse.
3. Inheritance: Classes can inherit characteristics from other classes, allowing for the creation of a hierarchy of classes that can reuse and extend existing functionality.
4. Polymorphism: This is the ability for a child class to take on many forms, allowing it to be treated as instances of its parent class.
### What is an Object?
An object is an instance of a class. It is a specific realization of the general template provided by the class. When you create an object, you are creating a unique entity that has its own state (data) and behavior (methods).
#### Key Features of an Object:
1. State: Each object maintains its own state. For example, if you have a `Dog` class, each `Dog` object may have different values for its attributes like `color`, `name`, and `breed`.
2. Behavior: Objects can perform actions defined by their class. Continuing with the `Dog` example, behaviors could include `wagging the tail`, `barking`, and `eating`.
3. Identity: Each object has a unique identity, which distinguishes it from other objects, even if they are of the same class.
### The Relationship Between Classes and Objects:
- Creation: Classes are used to create objects. You can think of a class as a cookie cutter, and objects are the cookies it produces.
- Inheritance: Objects inherit properties and behaviors from their class. If a `Dog` class has a `bark` method, every `Dog` object will have access to that method.
- Interaction: Objects interact with each other through messages, which are essentially method calls.
### Example:
Let's consider a simple example to illustrate the concept further. Imagine a `Vehicle` class with attributes like `make`, `model`, and `year`, and behaviors like `start`, `stop`, and `honk`.
```java
public class Vehicle {
String make;
String model;
int year;
public void start() {
// Code to start the vehicle
}
public void stop() {
// Code to stop the vehicle
}
public void honk() {
// Code to make the vehicle honk
}
}
```
Now, if you create an object from this class:
```java
Vehicle myCar = new Vehicle();
myCar.make = "Toyota";
myCar.model = "Corolla";
myCar.year = 2020;
```
Here, `myCar` is an instance of the `Vehicle` class. It has its own state (make, model, year) and can perform the behaviors defined in the class (start, stop, honk).
### Conclusion:
Understanding the distinction between classes and objects is crucial for grasping the principles of OOP. Classes are the blueprints that define the structure and behavior of objects, while objects are the tangible entities that are created from these blueprints. They encapsulate data and functionality, allowing for the creation of complex systems that are easier to manage and extend.
2024-05-12 17:45:14
reply(1)
Helpful(1122)
Helpful
Helpful(2)
Studied at the University of Buenos Aires, Lives in Buenos Aires, Argentina.
Object ? Objects have states and behaviors. Example: A dog has states - color, name, breed as well as behaviors -C wagging the tail, barking, eating. An object is an instance of a class. Class ? A class can be defined as a template/blueprint that describes the behavior/state that the object of its type support.
2023-06-15 03:56:36
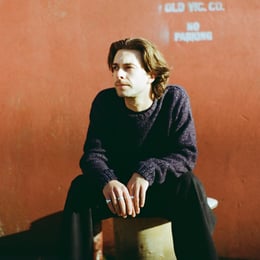
Amelia Lee
QuesHub.com delivers expert answers and knowledge to you.
Object ? Objects have states and behaviors. Example: A dog has states - color, name, breed as well as behaviors -C wagging the tail, barking, eating. An object is an instance of a class. Class ? A class can be defined as a template/blueprint that describes the behavior/state that the object of its type support.