Can an abstract class have a final method?
I'll answer
Earn 20 gold coins for an accepted answer.20
Earn 20 gold coins for an accepted answer.
40more
40more

Emily Lee
Studied at University of California, Berkeley, Lives in Berkeley, CA
Hello, I'm an expert in object-oriented programming and design patterns. I'm here to help you understand the intricacies of abstract classes and final methods.
An abstract class in Java is a class that cannot be instantiated on its own and is often used as a base class for other classes. It typically contains abstract methods, which are methods without an implementation that must be provided by any subclass. The purpose of an abstract class is to provide a common definition for a set of related classes and to enforce a certain structure or behavior.
Now, let's talk about final methods. A final method is a method that cannot be overridden by subclasses. Once a method is declared as final, it sets the last word on what that method does. Any subclass that extends the class containing the final method cannot provide its own implementation of that method.
Now, coming to your question, can an abstract class have a final method? The answer is yes, an abstract class can indeed have a final method. However, there's a catch. If a method in an abstract class is declared final, it means that subclasses cannot override that method. But since the abstract class itself cannot be instantiated, the final method must still be implemented by the subclass. This might seem contradictory at first, but it makes sense when you consider the purpose of each construct.
The abstract class is meant to provide a common interface and behavior that all subclasses must adhere to. By declaring a method as final, you're enforcing a certain implementation or behavior that cannot be changed by subclasses. This is useful when you want to ensure that a particular method's behavior remains consistent across all subclasses.
On the other hand, the abstract methods in an abstract class are meant to be implemented by the subclasses. They provide a contract that the subclasses must fulfill. By not providing an implementation for these methods in the abstract class, you allow the subclasses to provide their own specific behavior.
An example of this is the template method pattern. In this design pattern, you have an abstract class with both abstract and final methods. The final methods implement certain steps of an algorithm, while the abstract methods define the steps that subclasses must implement. This allows the abstract class to define the overall structure of an algorithm, while allowing subclasses to provide the specific details.
Here's a simple example to illustrate this:
```java
public abstract class Animal {
public final void breathe() {
System.out.println("This animal breathes air.");
}
public abstract void makeSound();
}
public class Dog extends Animal {
public void makeSound() {
System.out.println("Woof!");
}
}
```
In this example, the `Animal` class is an abstract class with a final method `breathe()` and an abstract method `makeSound()`. The `Dog` class is a subclass of `Animal` that provides its own implementation of the `makeSound()` method. However, it cannot override the `breathe()` method because it's final.
So, in conclusion, while it might seem counterintuitive, an abstract class can have a final method. It's a powerful way to enforce certain behaviors in subclasses while still allowing them to provide their own specific implementations for other methods.
An abstract class in Java is a class that cannot be instantiated on its own and is often used as a base class for other classes. It typically contains abstract methods, which are methods without an implementation that must be provided by any subclass. The purpose of an abstract class is to provide a common definition for a set of related classes and to enforce a certain structure or behavior.
Now, let's talk about final methods. A final method is a method that cannot be overridden by subclasses. Once a method is declared as final, it sets the last word on what that method does. Any subclass that extends the class containing the final method cannot provide its own implementation of that method.
Now, coming to your question, can an abstract class have a final method? The answer is yes, an abstract class can indeed have a final method. However, there's a catch. If a method in an abstract class is declared final, it means that subclasses cannot override that method. But since the abstract class itself cannot be instantiated, the final method must still be implemented by the subclass. This might seem contradictory at first, but it makes sense when you consider the purpose of each construct.
The abstract class is meant to provide a common interface and behavior that all subclasses must adhere to. By declaring a method as final, you're enforcing a certain implementation or behavior that cannot be changed by subclasses. This is useful when you want to ensure that a particular method's behavior remains consistent across all subclasses.
On the other hand, the abstract methods in an abstract class are meant to be implemented by the subclasses. They provide a contract that the subclasses must fulfill. By not providing an implementation for these methods in the abstract class, you allow the subclasses to provide their own specific behavior.
An example of this is the template method pattern. In this design pattern, you have an abstract class with both abstract and final methods. The final methods implement certain steps of an algorithm, while the abstract methods define the steps that subclasses must implement. This allows the abstract class to define the overall structure of an algorithm, while allowing subclasses to provide the specific details.
Here's a simple example to illustrate this:
```java
public abstract class Animal {
public final void breathe() {
System.out.println("This animal breathes air.");
}
public abstract void makeSound();
}
public class Dog extends Animal {
public void makeSound() {
System.out.println("Woof!");
}
}
```
In this example, the `Animal` class is an abstract class with a final method `breathe()` and an abstract method `makeSound()`. The `Dog` class is a subclass of `Animal` that provides its own implementation of the `makeSound()` method. However, it cannot override the `breathe()` method because it's final.
So, in conclusion, while it might seem counterintuitive, an abstract class can have a final method. It's a powerful way to enforce certain behaviors in subclasses while still allowing them to provide their own specific implementations for other methods.
2024-05-12 23:21:25
reply(1)
Helpful(1122)
Helpful
Helpful(2)
Studied at University of California, Los Angeles (UCLA), Lives in Los Angeles, CA
Yes, those methods cannot be overriden in subclasses. An example of that is the template method pattern... Of course, it means you can subclass it, but you cannot override that particular method. ... So we can declare a method as final in Abstract class, and it will be over ridden in subclass.Nov 12, 2011
2023-06-15 02:35:31
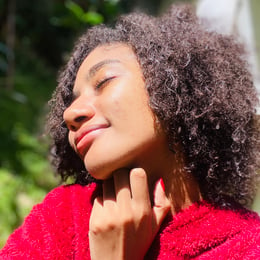
Harper Lee
QuesHub.com delivers expert answers and knowledge to you.
Yes, those methods cannot be overriden in subclasses. An example of that is the template method pattern... Of course, it means you can subclass it, but you cannot override that particular method. ... So we can declare a method as final in Abstract class, and it will be over ridden in subclass.Nov 12, 2011