What is dynamic in C#?
I'll answer
Earn 20 gold coins for an accepted answer.20
Earn 20 gold coins for an accepted answer.
40more
40more

Isabella Hall
Studied at the University of Seoul, Lives in Seoul, South Korea.
As a domain expert in programming with a focus on C#, I'm often asked about various aspects of the language. Today, let's delve into the concept of the `dynamic` keyword in C#. This is a fascinating feature that was introduced with C# 4.0 and it has significant implications for how developers can interact with objects in their code.
The `dynamic` keyword in C# is a type specifier that tells the compiler to treat a variable as if it were of a dynamic type. This means that the operations on the variable are not resolved until runtime, which is in stark contrast to the static typing that C# is typically known for. The key benefit of using `dynamic` is the ability to work with objects whose types are not known at compile time, which is particularly useful when dealing with late-bound operations or when using libraries that expose a dynamic interface.
When you declare a variable as `dynamic`, you're essentially telling the compiler to defer the type checking for that variable until runtime. This allows you to call methods and access properties on objects without having to know their exact types beforehand. It's a powerful tool for scenarios where you're dealing with objects that are not strongly typed, such as when working with COM objects, dynamic data structures, or certain types of reflection.
However, with great power comes great responsibility. Using `dynamic` does come with trade-offs. One of the most significant is that you lose the benefits of compile-time type checking. This means that any type-related errors will not be caught until the code is executed, which can lead to runtime exceptions if the operations you're performing are not valid for the actual types at runtime.
Another consideration is performance. Because the type resolution is deferred until runtime, operations on `dynamic` types can be slower than their statically typed counterparts. The compiler has to generate more code to handle the dynamic dispatch, which can lead to less efficient execution.
Here's a simple example to illustrate the use of `dynamic`:
```csharp
dynamic d = "Hello, World!";
int length = d.Length; // This would cause a compile-time error if 'd' were not dynamic.
```
In the above example, we're assigning a string to a `dynamic` variable `d` and then calling a `Length` property on it. If `d` were not declared as `dynamic`, this would result in a compile-time error because a string does not have a `Length` property in C#. However, because `d` is `dynamic`, the compiler allows this and the actual resolution of the property occurs at runtime.
In conclusion, the `dynamic` keyword in C# is a powerful feature that allows for more flexible and dynamic programming. It is particularly useful when working with objects whose types are not known until runtime. However, developers should use it judiciously, being aware of the trade-offs in terms of type safety and potential performance implications.
Now, let's move on to the translation.
The `dynamic` keyword in C# is a type specifier that tells the compiler to treat a variable as if it were of a dynamic type. This means that the operations on the variable are not resolved until runtime, which is in stark contrast to the static typing that C# is typically known for. The key benefit of using `dynamic` is the ability to work with objects whose types are not known at compile time, which is particularly useful when dealing with late-bound operations or when using libraries that expose a dynamic interface.
When you declare a variable as `dynamic`, you're essentially telling the compiler to defer the type checking for that variable until runtime. This allows you to call methods and access properties on objects without having to know their exact types beforehand. It's a powerful tool for scenarios where you're dealing with objects that are not strongly typed, such as when working with COM objects, dynamic data structures, or certain types of reflection.
However, with great power comes great responsibility. Using `dynamic` does come with trade-offs. One of the most significant is that you lose the benefits of compile-time type checking. This means that any type-related errors will not be caught until the code is executed, which can lead to runtime exceptions if the operations you're performing are not valid for the actual types at runtime.
Another consideration is performance. Because the type resolution is deferred until runtime, operations on `dynamic` types can be slower than their statically typed counterparts. The compiler has to generate more code to handle the dynamic dispatch, which can lead to less efficient execution.
Here's a simple example to illustrate the use of `dynamic`:
```csharp
dynamic d = "Hello, World!";
int length = d.Length; // This would cause a compile-time error if 'd' were not dynamic.
```
In the above example, we're assigning a string to a `dynamic` variable `d` and then calling a `Length` property on it. If `d` were not declared as `dynamic`, this would result in a compile-time error because a string does not have a `Length` property in C#. However, because `d` is `dynamic`, the compiler allows this and the actual resolution of the property occurs at runtime.
In conclusion, the `dynamic` keyword in C# is a powerful feature that allows for more flexible and dynamic programming. It is particularly useful when working with objects whose types are not known until runtime. However, developers should use it judiciously, being aware of the trade-offs in terms of type safety and potential performance implications.
Now, let's move on to the translation.
2024-05-13 00:55:28
reply(1)
Helpful(1122)
Helpful
Helpful(2)
Works at the International Fund for Agricultural Development, Lives in Rome, Italy.
The dynamic keyword is new to C# 4.0, and is used to tell the compiler that a variable's type can change or that it is not known until runtime. Think of it as being able to interact with an Object without having to cast it. ... Now, of course, when you are using a dynamic variable, you are giving up compiler type checking.
2023-06-12 02:02:27
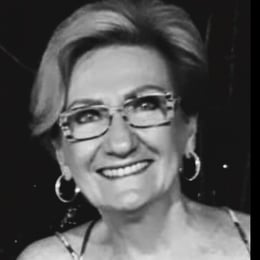
Amelia Gonzalez
QuesHub.com delivers expert answers and knowledge to you.
The dynamic keyword is new to C# 4.0, and is used to tell the compiler that a variable's type can change or that it is not known until runtime. Think of it as being able to interact with an Object without having to cast it. ... Now, of course, when you are using a dynamic variable, you are giving up compiler type checking.