What is a predicate in C++ 2024?
I'll answer
Earn 20 gold coins for an accepted answer.20
Earn 20 gold coins for an accepted answer.
40more
40more
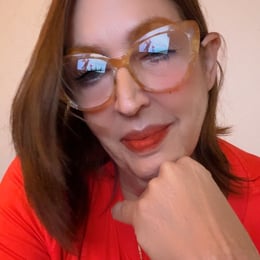
Isabella Lewis
Studied at the University of Sydney, Lives in Sydney, Australia.
As a seasoned expert in the field of computer science, particularly with a focus on C++ programming, I'm well-versed in the intricacies of the language and its various constructs. Let's delve into the concept of a predicate in C++.
In C++, a predicate is a function or a functor that returns a Boolean value, which is either `true` or `false`. This is a fundamental concept used in various algorithms and operations where a condition needs to be evaluated. Predicates are often used with standard library algorithms that perform operations based on a condition, such as `std::find_if`, `std::sort`, and `std::remove_if`.
The term "predicate" originates from mathematical logic, where it refers to a statement that can be true or false. In C++, this concept is embodied in functions or functors that encapsulate a condition.
### Predicate as a Function
A predicate can be implemented as a plain function. For instance, consider a simple function that checks if a number is even:
```cpp
bool isEven(int number) {
return number % 2 == 0;
}
```
This function can be used as a predicate with algorithms that accept a predicate function.
### Predicate as a Functor
A functor is an object that can be invoked as if it were a function. It is essentially a class with an overloaded `operator()` that defines the predicate logic. Here's an example of a functor that performs the same check as the `isEven` function:
```cpp
class IsEven {
public:
bool operator()(int number) const {
return number % 2 == 0;
}
};
```
You can use this functor with standard algorithms by passing an instance of `IsEven` as the predicate.
### Predicates and Standard Library Algorithms
The C++ Standard Library makes extensive use of predicates in its algorithms. For example, `std::find_if` can be used to find the first element in a container that satisfies a given predicate:
```cpp
#include <algorithm>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
auto it = std::find_if(numbers.begin(), numbers.end(), isEven);
if (it != numbers.end()) {
std::cout << "Found even number: " << *it << std::endl;
}
return 0;
}
```
In this example, `isEven` is a predicate that is used to find the first even number in the `numbers` vector.
### Predicates and Lambda Expressions
With the introduction of lambda expressions in C++11, writing predicates has become even more concise. A lambda expression can be used directly as a predicate:
```cpp
auto it = std::find_if(numbers.begin(), numbers.end(), [](int number) {
return number % 2 == 0;
});
```
This lambda expression captures the essence of the `isEven` function or functor but is written in a more compact form.
### Importance of Predicates
Predicates are crucial in functional programming paradigms and are extensively used in C++ for their flexibility and expressiveness. They allow for writing clean, reusable, and maintainable code by separating the condition-checking logic from the algorithm that performs the operation.
In summary, predicates in C++ are powerful tools for condition-based operations, enhancing the language's capability to handle complex logic in a clean and efficient manner.
In C++, a predicate is a function or a functor that returns a Boolean value, which is either `true` or `false`. This is a fundamental concept used in various algorithms and operations where a condition needs to be evaluated. Predicates are often used with standard library algorithms that perform operations based on a condition, such as `std::find_if`, `std::sort`, and `std::remove_if`.
The term "predicate" originates from mathematical logic, where it refers to a statement that can be true or false. In C++, this concept is embodied in functions or functors that encapsulate a condition.
### Predicate as a Function
A predicate can be implemented as a plain function. For instance, consider a simple function that checks if a number is even:
```cpp
bool isEven(int number) {
return number % 2 == 0;
}
```
This function can be used as a predicate with algorithms that accept a predicate function.
### Predicate as a Functor
A functor is an object that can be invoked as if it were a function. It is essentially a class with an overloaded `operator()` that defines the predicate logic. Here's an example of a functor that performs the same check as the `isEven` function:
```cpp
class IsEven {
public:
bool operator()(int number) const {
return number % 2 == 0;
}
};
```
You can use this functor with standard algorithms by passing an instance of `IsEven` as the predicate.
### Predicates and Standard Library Algorithms
The C++ Standard Library makes extensive use of predicates in its algorithms. For example, `std::find_if` can be used to find the first element in a container that satisfies a given predicate:
```cpp
#include <algorithm>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
auto it = std::find_if(numbers.begin(), numbers.end(), isEven);
if (it != numbers.end()) {
std::cout << "Found even number: " << *it << std::endl;
}
return 0;
}
```
In this example, `isEven` is a predicate that is used to find the first even number in the `numbers` vector.
### Predicates and Lambda Expressions
With the introduction of lambda expressions in C++11, writing predicates has become even more concise. A lambda expression can be used directly as a predicate:
```cpp
auto it = std::find_if(numbers.begin(), numbers.end(), [](int number) {
return number % 2 == 0;
});
```
This lambda expression captures the essence of the `isEven` function or functor but is written in a more compact form.
### Importance of Predicates
Predicates are crucial in functional programming paradigms and are extensively used in C++ for their flexibility and expressiveness. They allow for writing clean, reusable, and maintainable code by separating the condition-checking logic from the algorithm that performs the operation.
In summary, predicates in C++ are powerful tools for condition-based operations, enhancing the language's capability to handle complex logic in a clean and efficient manner.
2024-06-16 16:46:41
reply(1)
Helpful(1122)
Helpful
Helpful(2)
Works at Netflix, Lives in Los Gatos, CA
Predicates are functions that return a Boolean value (or something that can be implicitly converted to bool). In other words, a predicate class is a functor class whose operator() function is a predicate, i.e., its operator() returns true or false. ... A unary function that returns a bool value is a predicate.
2023-06-14 02:02:26

Cameron Powell
QuesHub.com delivers expert answers and knowledge to you.
Predicates are functions that return a Boolean value (or something that can be implicitly converted to bool). In other words, a predicate class is a functor class whose operator() function is a predicate, i.e., its operator() returns true or false. ... A unary function that returns a bool value is a predicate.