What is a predicate in C#?
I'll answer
Earn 20 gold coins for an accepted answer.20
Earn 20 gold coins for an accepted answer.
40more
40more
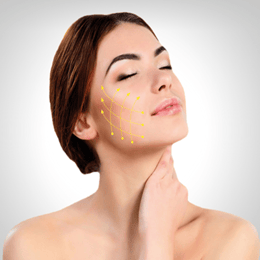
Charlotte Wilson
Studied at the University of Johannesburg, Lives in Johannesburg, South Africa.
Hi there! I'm an expert in programming languages with a deep understanding of C#. Let's dive into the concept of a predicate in C#.
A predicate in C# is a delegate that represents a method that takes one or more parameters and returns a Boolean value. It is commonly used in LINQ queries to filter a collection based on a condition. The Predicate class is defined in the System namespace and provides methods to create and work with predicates.
Here's a step-by-step explanation of how a predicate works in C#:
1. Declaration: A predicate is declared as a delegate type, which specifies the signature of the method it represents. The delegate type is defined using the `delegate` keyword followed by the return type (in this case, `bool`) and a parameter list.
```csharp
public delegate bool Predicate<T>(T obj);
```
This declares a generic predicate delegate that takes an object of type `T` and returns a `bool`.
2. Instantiation: A predicate can be instantiated using an anonymous method, a lambda expression, or a method group. Here are some examples:
```csharp
// Anonymous method
Predicate<int> p1 = delegate(int x) { return x > 10; };
// Lambda expression
Predicate<string> p2 = x => x.StartsWith("A");
// Method group
Predicate<DateTime> p3 = IsAfterYear2000;
bool IsAfterYear2000(DateTime dt) => dt.Year > 2000;
```
3. Usage: Once instantiated, a predicate can be used in various methods and operators that accept a predicate as a parameter. Some common examples are:
- `Find`: Searches for an element in a collection that matches the predicate.
- `FindAll`: Returns all elements in a collection that match the predicate.
- `Where`: Filters a collection based on the predicate in a LINQ query.
Here's an example of using a predicate with the `Find` method:
```csharp
List<int> numbers = new List<int> { 1, 2, 3, 4, 5, 6, 7, 8, 9, 10 };
Predicate<int> greaterThan5 = x => x > 5;
int result = numbers.Find(greaterThan5);
Console.WriteLine(result); // Output: 6
```
In this example, we define a predicate `greaterThan5` that checks if a number is greater than 5. We then use the `Find` method to find the first number in the `numbers` list that satisfies this condition.
4. Inline Delegates: As mentioned earlier, C# supports inline delegates, which allow you to create an anonymous method and assign it to a predicate variable in a single line of code. This makes it easy to create predicates on the fly without having to define a separate method or lambda expression. Here's an example:
```csharp
Predicate<double> hasPositiveExponent = delegate(double num) { return Math.Pow(num, 2) > 0; };
```
This creates a predicate that checks if the square of a number has a positive exponent.
5. Performance Considerations: While predicates provide a flexible and expressive way to filter collections, it's important to be mindful of performance. Using complex predicates with large collections can lead to performance bottlenecks. In such cases, it may be more efficient to use other filtering mechanisms or optimize the predicate logic.
In summary, a predicate in C# is a powerful tool for filtering and querying collections based on conditions. By understanding how to declare, instantiate, and use predicates, you can write more expressive and efficient code in your C# applications.
A predicate in C# is a delegate that represents a method that takes one or more parameters and returns a Boolean value. It is commonly used in LINQ queries to filter a collection based on a condition. The Predicate class is defined in the System namespace and provides methods to create and work with predicates.
Here's a step-by-step explanation of how a predicate works in C#:
1. Declaration: A predicate is declared as a delegate type, which specifies the signature of the method it represents. The delegate type is defined using the `delegate` keyword followed by the return type (in this case, `bool`) and a parameter list.
```csharp
public delegate bool Predicate<T>(T obj);
```
This declares a generic predicate delegate that takes an object of type `T` and returns a `bool`.
2. Instantiation: A predicate can be instantiated using an anonymous method, a lambda expression, or a method group. Here are some examples:
```csharp
// Anonymous method
Predicate<int> p1 = delegate(int x) { return x > 10; };
// Lambda expression
Predicate<string> p2 = x => x.StartsWith("A");
// Method group
Predicate<DateTime> p3 = IsAfterYear2000;
bool IsAfterYear2000(DateTime dt) => dt.Year > 2000;
```
3. Usage: Once instantiated, a predicate can be used in various methods and operators that accept a predicate as a parameter. Some common examples are:
- `Find`: Searches for an element in a collection that matches the predicate.
- `FindAll`: Returns all elements in a collection that match the predicate.
- `Where`: Filters a collection based on the predicate in a LINQ query.
Here's an example of using a predicate with the `Find` method:
```csharp
List<int> numbers = new List<int> { 1, 2, 3, 4, 5, 6, 7, 8, 9, 10 };
Predicate<int> greaterThan5 = x => x > 5;
int result = numbers.Find(greaterThan5);
Console.WriteLine(result); // Output: 6
```
In this example, we define a predicate `greaterThan5` that checks if a number is greater than 5. We then use the `Find` method to find the first number in the `numbers` list that satisfies this condition.
4. Inline Delegates: As mentioned earlier, C# supports inline delegates, which allow you to create an anonymous method and assign it to a predicate variable in a single line of code. This makes it easy to create predicates on the fly without having to define a separate method or lambda expression. Here's an example:
```csharp
Predicate<double> hasPositiveExponent = delegate(double num) { return Math.Pow(num, 2) > 0; };
```
This creates a predicate that checks if the square of a number has a positive exponent.
5. Performance Considerations: While predicates provide a flexible and expressive way to filter collections, it's important to be mindful of performance. Using complex predicates with large collections can lead to performance bottlenecks. In such cases, it may be more efficient to use other filtering mechanisms or optimize the predicate logic.
In summary, a predicate in C# is a powerful tool for filtering and querying collections based on conditions. By understanding how to declare, instantiate, and use predicates, you can write more expressive and efficient code in your C# applications.
2024-05-13 16:59:00
reply(1)
Helpful(1122)
Helpful
Helpful(2)
Studied at University of California, Berkeley, Lives in Berkeley, CA
A predicate is also a delegate like Func and Action delegates. It represents a method that contains a set of criteria and checks whether the passed parameter meets those criteria or not. A predicate delegate methods must take one input parameter and it then returns a boolean value - true or false.
2023-06-15 02:02:25
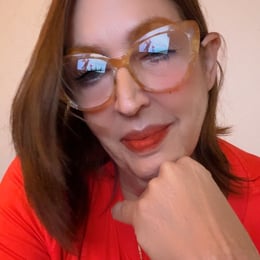
Isabella Lewis
QuesHub.com delivers expert answers and knowledge to you.
A predicate is also a delegate like Func and Action delegates. It represents a method that contains a set of criteria and checks whether the passed parameter meets those criteria or not. A predicate delegate methods must take one input parameter and it then returns a boolean value - true or false.